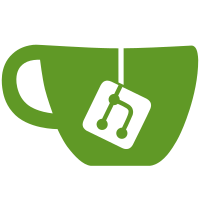
Many scripts compare actual and expected output using "diff -u". This is nicer than "cmp" because the output shows how the two differ. However, not all versions of diff understand -u, leading to unnecessary test failure. This adds a test_cmp function to the test scripts and switches all "diff -u" invocations to use it. The function uses the contents of "$GIT_TEST_CMP" to compare its arguments; the default is "diff -u". On systems with a less-capable diff, you can do: GIT_TEST_CMP=cmp make test Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
140 lines
2.5 KiB
Bash
Executable File
140 lines
2.5 KiB
Bash
Executable File
#!/bin/sh
|
|
#
|
|
# Copyright (c) 2006 Junio C Hamano
|
|
#
|
|
|
|
test_description='quoted output'
|
|
|
|
. ./test-lib.sh
|
|
|
|
P1='pathname with HT'
|
|
: >"$P1" 2>&1 && test -f "$P1" && rm -f "$P1" || {
|
|
echo >&2 'Filesystem does not support HT in names'
|
|
test_done
|
|
}
|
|
|
|
FN='濱野'
|
|
GN='純'
|
|
HT=' '
|
|
LF='
|
|
'
|
|
DQ='"'
|
|
|
|
echo foo > "Name and an${HT}HT"
|
|
test -f "Name and an${HT}HT" || {
|
|
# since FAT/NTFS does not allow tabs in filenames, skip this test
|
|
say 'Your filesystem does not allow tabs in filenames, test skipped.'
|
|
test_done
|
|
}
|
|
|
|
for_each_name () {
|
|
for name in \
|
|
Name "Name and a${LF}LF" "Name and an${HT}HT" "Name${DQ}" \
|
|
"$FN$HT$GN" "$FN$LF$GN" "$FN $GN" "$FN$GN" "$FN$DQ$GN" \
|
|
"With SP in it"
|
|
do
|
|
eval "$1"
|
|
done
|
|
}
|
|
|
|
test_expect_success setup '
|
|
|
|
for_each_name "echo initial >\"\$name\""
|
|
git add . &&
|
|
git commit -q -m Initial &&
|
|
|
|
for_each_name "echo second >\"\$name\"" &&
|
|
git commit -a -m Second
|
|
|
|
for_each_name "echo modified >\"\$name\""
|
|
|
|
'
|
|
|
|
cat >expect.quoted <<\EOF
|
|
Name
|
|
"Name and a\nLF"
|
|
"Name and an\tHT"
|
|
"Name\""
|
|
With SP in it
|
|
"\346\277\261\351\207\216\t\347\264\224"
|
|
"\346\277\261\351\207\216\n\347\264\224"
|
|
"\346\277\261\351\207\216 \347\264\224"
|
|
"\346\277\261\351\207\216\"\347\264\224"
|
|
"\346\277\261\351\207\216\347\264\224"
|
|
EOF
|
|
|
|
cat >expect.raw <<\EOF
|
|
Name
|
|
"Name and a\nLF"
|
|
"Name and an\tHT"
|
|
"Name\""
|
|
With SP in it
|
|
"濱野\t純"
|
|
"濱野\n純"
|
|
濱野 純
|
|
"濱野\"純"
|
|
濱野純
|
|
EOF
|
|
|
|
test_expect_success 'check fully quoted output from ls-files' '
|
|
|
|
git ls-files >current && test_cmp expect.quoted current
|
|
|
|
'
|
|
|
|
test_expect_success 'check fully quoted output from diff-files' '
|
|
|
|
git diff --name-only >current &&
|
|
test_cmp expect.quoted current
|
|
|
|
'
|
|
|
|
test_expect_success 'check fully quoted output from diff-index' '
|
|
|
|
git diff --name-only HEAD >current &&
|
|
test_cmp expect.quoted current
|
|
|
|
'
|
|
|
|
test_expect_success 'check fully quoted output from diff-tree' '
|
|
|
|
git diff --name-only HEAD^ HEAD >current &&
|
|
test_cmp expect.quoted current
|
|
|
|
'
|
|
|
|
test_expect_success 'setting core.quotepath' '
|
|
|
|
git config --bool core.quotepath false
|
|
|
|
'
|
|
|
|
test_expect_success 'check fully quoted output from ls-files' '
|
|
|
|
git ls-files >current && test_cmp expect.raw current
|
|
|
|
'
|
|
|
|
test_expect_success 'check fully quoted output from diff-files' '
|
|
|
|
git diff --name-only >current &&
|
|
test_cmp expect.raw current
|
|
|
|
'
|
|
|
|
test_expect_success 'check fully quoted output from diff-index' '
|
|
|
|
git diff --name-only HEAD >current &&
|
|
test_cmp expect.raw current
|
|
|
|
'
|
|
|
|
test_expect_success 'check fully quoted output from diff-tree' '
|
|
|
|
git diff --name-only HEAD^ HEAD >current &&
|
|
test_cmp expect.raw current
|
|
|
|
'
|
|
|
|
test_done
|