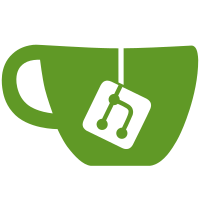
If you have whitespace errors in lines you've introduced, it can be convenient to be able to jump directly to them for fixing. You can't quite use "git jump diff" for this, because though it passes arbitrary options to "git diff", it expects to see an actual unified diff in the output. Whereas "git diff --check" actually produces lines that look like compiler quickfix lines already, meaning we just need to run it and feed the output directly to the editor. Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
76 lines
1.4 KiB
Bash
Executable File
76 lines
1.4 KiB
Bash
Executable File
#!/bin/sh
|
|
|
|
usage() {
|
|
cat <<\EOF
|
|
usage: git jump <mode> [<args>]
|
|
|
|
Jump to interesting elements in an editor.
|
|
The <mode> parameter is one of:
|
|
|
|
diff: elements are diff hunks. Arguments are given to diff.
|
|
|
|
merge: elements are merge conflicts. Arguments are ignored.
|
|
|
|
grep: elements are grep hits. Arguments are given to grep.
|
|
|
|
ws: elements are whitespace errors. Arguments are given to diff --check.
|
|
EOF
|
|
}
|
|
|
|
open_editor() {
|
|
editor=`git var GIT_EDITOR`
|
|
eval "$editor -q \$1"
|
|
}
|
|
|
|
mode_diff() {
|
|
git diff --no-prefix --relative "$@" |
|
|
perl -ne '
|
|
if (m{^\+\+\+ (.*)}) { $file = $1; next }
|
|
defined($file) or next;
|
|
if (m/^@@ .*?\+(\d+)/) { $line = $1; next }
|
|
defined($line) or next;
|
|
if (/^ /) { $line++; next }
|
|
if (/^[-+]\s*(.*)/) {
|
|
print "$file:$line: $1\n";
|
|
$line = undef;
|
|
}
|
|
'
|
|
}
|
|
|
|
mode_merge() {
|
|
git ls-files -u |
|
|
perl -pe 's/^.*?\t//' |
|
|
sort -u |
|
|
while IFS= read fn; do
|
|
grep -Hn '^<<<<<<<' "$fn"
|
|
done
|
|
}
|
|
|
|
# Grep -n generates nice quickfix-looking lines by itself,
|
|
# but let's clean up extra whitespace, so they look better if the
|
|
# editor shows them to us in the status bar.
|
|
mode_grep() {
|
|
git grep -n "$@" |
|
|
perl -pe '
|
|
s/[ \t]+/ /g;
|
|
s/^ *//;
|
|
'
|
|
}
|
|
|
|
mode_ws() {
|
|
git diff --check "$@"
|
|
}
|
|
|
|
if test $# -lt 1; then
|
|
usage >&2
|
|
exit 1
|
|
fi
|
|
mode=$1; shift
|
|
|
|
trap 'rm -f "$tmp"' 0 1 2 3 15
|
|
tmp=`mktemp -t git-jump.XXXXXX` || exit 1
|
|
type "mode_$mode" >/dev/null 2>&1 || { usage >&2; exit 1; }
|
|
"mode_$mode" "$@" >"$tmp"
|
|
test -s "$tmp" || exit 0
|
|
open_editor "$tmp"
|