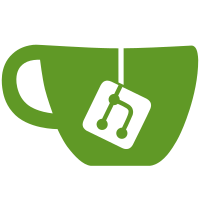
test-prio-queue.c doesn't check the return value of malloc, and could segfault. It's unlikely for this to matter in practice; it's a small allocation, and this code isn't even installed alongside the rest of Git. But let's use xmalloc(), which makes auditing for other accidental uses of bare malloc() easier. Reported-by: 王健强 <jianqiang.wang@securitygossip.com> Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
51 lines
974 B
C
51 lines
974 B
C
#include "test-tool.h"
|
|
#include "cache.h"
|
|
#include "prio-queue.h"
|
|
|
|
static int intcmp(const void *va, const void *vb, void *data)
|
|
{
|
|
const int *a = va, *b = vb;
|
|
return *a - *b;
|
|
}
|
|
|
|
static void show(int *v)
|
|
{
|
|
if (!v)
|
|
printf("NULL\n");
|
|
else
|
|
printf("%d\n", *v);
|
|
free(v);
|
|
}
|
|
|
|
int cmd__prio_queue(int argc, const char **argv)
|
|
{
|
|
struct prio_queue pq = { intcmp };
|
|
|
|
while (*++argv) {
|
|
if (!strcmp(*argv, "get")) {
|
|
void *peek = prio_queue_peek(&pq);
|
|
void *get = prio_queue_get(&pq);
|
|
if (peek != get)
|
|
BUG("peek and get results do not match");
|
|
show(get);
|
|
} else if (!strcmp(*argv, "dump")) {
|
|
void *peek;
|
|
void *get;
|
|
while ((peek = prio_queue_peek(&pq))) {
|
|
get = prio_queue_get(&pq);
|
|
if (peek != get)
|
|
BUG("peek and get results do not match");
|
|
show(get);
|
|
}
|
|
} else if (!strcmp(*argv, "stack")) {
|
|
pq.compare = NULL;
|
|
} else {
|
|
int *v = xmalloc(sizeof(*v));
|
|
*v = atoi(*argv);
|
|
prio_queue_put(&pq, v);
|
|
}
|
|
}
|
|
|
|
return 0;
|
|
}
|