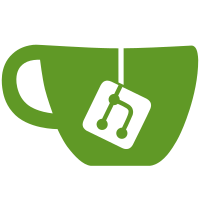
When a user fetches: - at least one up-to-date ref and at least one non-up-to-date ref, - using HTTP with protocol v0 (or something else that uses the fetch command of a remote helper) some refs might not be updated after the fetch. This bug was introduced in commit989b8c4452
("fetch-pack: put shallow info in output parameter", 2018-06-28) which allowed transports to report the refs that they have fetched in a new out-parameter "fetched_refs". If they do so, transport_fetch_refs() makes this information available to its caller. Users of "fetched_refs" rely on the following 3 properties: (1) it is the complete list of refs that was passed to transport_fetch_refs(), (2) it has shallow information (REF_STATUS_REJECT_SHALLOW set if relevant), and (3) it has updated OIDs if ref-in-want was used (introduced after989b8c4452
). In an effort to satisfy (1), whenever transport_fetch_refs() filters the refs sent to the transport, it re-adds the filtered refs to whatever the transport supplies before returning it to the user. However, the implementation in989b8c4452
unconditionally re-adds the filtered refs without checking if the transport refrained from reporting anything in "fetched_refs" (which it is allowed to do), resulting in an incomplete list, no longer satisfying (1). An earlier effort to resolve this [1] solved the issue by readding the filtered refs only if the transport did not refrain from reporting in "fetched_refs", but after further discussion, it seems that the better solution is to revert the API change that introduced "fetched_refs". This API change was first suggested as part of a ref-in-want implementation that allowed for ref patterns and, thus, there could be drastic differences between the input refs and the refs actually fetched [2]; we eventually decided to only allow exact ref names, but this API change remained even though its necessity was decreased. Therefore, revert this API change by reverting commit989b8c4452
, and make receive_wanted_refs() update the OIDs in the sought array (like how update_shallow() updates shallow information in the sought array) instead. A test is also included to show that the user-visible bug discussed at the beginning of this commit message no longer exists. [1] https://public-inbox.org/git/20180801171806.GA122458@google.com/ [2] https://public-inbox.org/git/86a128c5fb710a41791e7183207c4d64889f9307.1485381677.git.jonathantanmy@google.com/ Signed-off-by: Jonathan Tan <jonathantanmy@google.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
46 lines
1.2 KiB
C
46 lines
1.2 KiB
C
#include "cache.h"
|
|
#include "packfile.h"
|
|
#include "pkt-line.h"
|
|
#include "strbuf.h"
|
|
#include "transport.h"
|
|
#include "fetch-object.h"
|
|
|
|
static void fetch_refs(const char *remote_name, struct ref *ref)
|
|
{
|
|
struct remote *remote;
|
|
struct transport *transport;
|
|
int original_fetch_if_missing = fetch_if_missing;
|
|
|
|
fetch_if_missing = 0;
|
|
remote = remote_get(remote_name);
|
|
if (!remote->url[0])
|
|
die(_("Remote with no URL"));
|
|
transport = transport_get(remote, remote->url[0]);
|
|
|
|
transport_set_option(transport, TRANS_OPT_FROM_PROMISOR, "1");
|
|
transport_set_option(transport, TRANS_OPT_NO_DEPENDENTS, "1");
|
|
transport_fetch_refs(transport, ref);
|
|
fetch_if_missing = original_fetch_if_missing;
|
|
}
|
|
|
|
void fetch_object(const char *remote_name, const unsigned char *sha1)
|
|
{
|
|
struct ref *ref = alloc_ref(sha1_to_hex(sha1));
|
|
hashcpy(ref->old_oid.hash, sha1);
|
|
fetch_refs(remote_name, ref);
|
|
}
|
|
|
|
void fetch_objects(const char *remote_name, const struct oid_array *to_fetch)
|
|
{
|
|
struct ref *ref = NULL;
|
|
int i;
|
|
|
|
for (i = 0; i < to_fetch->nr; i++) {
|
|
struct ref *new_ref = alloc_ref(oid_to_hex(&to_fetch->oid[i]));
|
|
oidcpy(&new_ref->old_oid, &to_fetch->oid[i]);
|
|
new_ref->next = ref;
|
|
ref = new_ref;
|
|
}
|
|
fetch_refs(remote_name, ref);
|
|
}
|