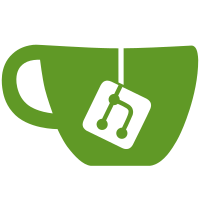
The confusion between "revs" vs "refs" caused us to not find the branch name, which in turn meant that we never switched the HEAD over to it.
52 lines
938 B
Bash
Executable File
52 lines
938 B
Bash
Executable File
#!/bin/sh
|
|
: ${GIT_DIR=.git}
|
|
old=$(git-rev-parse HEAD)
|
|
new=
|
|
force=
|
|
branch=
|
|
while [ "$#" != "0" ]; do
|
|
arg="$1"
|
|
shift
|
|
case "$arg" in
|
|
"-f")
|
|
force=1
|
|
;;
|
|
*)
|
|
rev=$(git-rev-parse "$arg")
|
|
if [ -z "$rev" ]; then
|
|
echo "unknown flag $arg"
|
|
exit 1
|
|
fi
|
|
if [ "$new" ]; then
|
|
echo "Multiple revisions?"
|
|
exit 1
|
|
fi
|
|
new="$rev"
|
|
if [ -f "$GIT_DIR/refs/heads/$arg" ]; then
|
|
branch="$arg"
|
|
fi
|
|
;;
|
|
esac
|
|
i=$(($i+1))
|
|
done
|
|
[ -z "$new" ] && new=$old
|
|
|
|
if [ "$force" ]
|
|
then
|
|
git-read-tree --reset $new &&
|
|
git-checkout-cache -q -f -u -a
|
|
else
|
|
git-read-tree -m -u $old $new
|
|
fi
|
|
|
|
#
|
|
# Switch the HEAD pointer to the new branch if it we
|
|
# checked out a branch head, and remove any potential
|
|
# old MERGE_HEAD's (subsequent commits will clearly not
|
|
# be based on them, since we re-set the index)
|
|
#
|
|
if [ "$?" -eq 0 ]; then
|
|
[ "$branch" ] && ln -sf "refs/heads/$branch" "$GIT_DIR/HEAD"
|
|
rm -f "$GIT_DIR/MERGE_HEAD"
|
|
fi
|