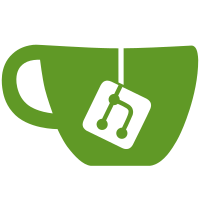
Our blame viewer has had a very fancy progress bar at the bottom of the window that shows the current status of the blame engine, which includes the number of lines completed as both a text and a graphical meter. I want to reuse this meter system in other places, such as during a branch switch where read-tree -v can give us a progress meter for any long-running operation. This change extracts the code and refactors it as a widget that we can take advantage of in locations other than in the blame viewer. Signed-off-by: Shawn O. Pearce <spearce@spearce.org>
77 lines
1.4 KiB
Tcl
77 lines
1.4 KiB
Tcl
# git-gui status bar mega-widget
|
|
# Copyright (C) 2007 Shawn Pearce
|
|
|
|
class status_bar {
|
|
|
|
field w ; # our own window path
|
|
field w_l ; # text widget we draw messages into
|
|
field w_c ; # canvas we draw a progress bar into
|
|
field status {}; # single line of text we show
|
|
field prefix {}; # text we format into status
|
|
field units {}; # unit of progress
|
|
|
|
constructor new {path} {
|
|
set w $path
|
|
set w_l $w.l
|
|
set w_c $w.c
|
|
|
|
frame $w \
|
|
-borderwidth 1 \
|
|
-relief sunken
|
|
label $w_l \
|
|
-textvariable @status \
|
|
-anchor w \
|
|
-justify left
|
|
pack $w_l -side left
|
|
|
|
bind $w <Destroy> [cb _delete %W]
|
|
return $this
|
|
}
|
|
|
|
method start {msg uds} {
|
|
if {[winfo exists $w_c]} {
|
|
$w_c coords bar 0 0 0 20
|
|
} else {
|
|
canvas $w_c \
|
|
-width 100 \
|
|
-height [expr {int([winfo reqheight $w_l] * 0.6)}] \
|
|
-borderwidth 1 \
|
|
-relief groove \
|
|
-highlightt 0
|
|
$w_c create rectangle 0 0 0 20 -tags bar -fill navy
|
|
pack $w_c -side right
|
|
}
|
|
|
|
set status $msg
|
|
set prefix $msg
|
|
set units $uds
|
|
}
|
|
|
|
method update {have total} {
|
|
set pdone 0
|
|
if {$total > 0} {
|
|
set pdone [expr {100 * $have / $total}]
|
|
}
|
|
|
|
set status [format "%s ... %i of %i %s (%2i%%)" \
|
|
$prefix $have $total $units $pdone]
|
|
$w_c coords bar 0 0 $pdone 20
|
|
}
|
|
|
|
method stop {msg} {
|
|
destroy $w_c
|
|
set status $msg
|
|
}
|
|
|
|
method show {msg} {
|
|
set status $msg
|
|
}
|
|
|
|
method _delete {current} {
|
|
if {$current eq $w} {
|
|
delete_this
|
|
}
|
|
}
|
|
|
|
}
|