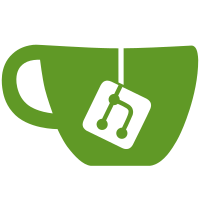
We ask to write 41 bytes and make sure that the return value is at least 41. This is the same "dangerous" pattern that was fixed in the prior commit (wherein a negative return value is promoted to unsigned), though it is not dangerous here because our "41" is a constant, not an unsigned variable. But we should convert it anyway to avoid modeling a dangerous construct. Signed-off-by: Jeff King <peff@peff.net> Signed-off-by: Junio C Hamano <gitster@pobox.com>
41 lines
959 B
C
41 lines
959 B
C
/*
|
|
* Copyright (c) 2005, 2006 Rene Scharfe
|
|
*/
|
|
#include "cache.h"
|
|
#include "commit.h"
|
|
#include "tar.h"
|
|
#include "builtin.h"
|
|
#include "quote.h"
|
|
|
|
static const char builtin_get_tar_commit_id_usage[] =
|
|
"git get-tar-commit-id";
|
|
|
|
/* ustar header + extended global header content */
|
|
#define RECORDSIZE (512)
|
|
#define HEADERSIZE (2 * RECORDSIZE)
|
|
|
|
int cmd_get_tar_commit_id(int argc, const char **argv, const char *prefix)
|
|
{
|
|
char buffer[HEADERSIZE];
|
|
struct ustar_header *header = (struct ustar_header *)buffer;
|
|
char *content = buffer + RECORDSIZE;
|
|
const char *comment;
|
|
ssize_t n;
|
|
|
|
if (argc != 1)
|
|
usage(builtin_get_tar_commit_id_usage);
|
|
|
|
n = read_in_full(0, buffer, HEADERSIZE);
|
|
if (n < HEADERSIZE)
|
|
die("git get-tar-commit-id: read error");
|
|
if (header->typeflag[0] != 'g')
|
|
return 1;
|
|
if (!skip_prefix(content, "52 comment=", &comment))
|
|
return 1;
|
|
|
|
if (write_in_full(1, comment, 41) < 0)
|
|
die_errno("git get-tar-commit-id: write error");
|
|
|
|
return 0;
|
|
}
|